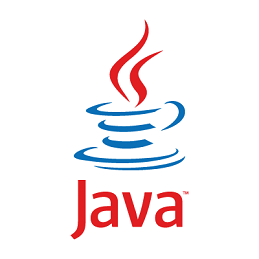
Java Platform, Standard Edition or Java SE is a widely used platform for development and deployment of portable code for desktop and server environments.Java SE uses the object-oriented Java programming language. It is part of the Java software platform family. Java SE defines a wide range of general purpose APIs – such as Java APIs for the Java Class Library – and also includes the Java Language Specification and the Java Virtual Machine Specification.One of the most well-known implementations of Java SE is Oracle Corporation's Java Development Kit (JDK).
Core Java
Java Fundamentals
Introduction to Java language, Advantages of JAVA, Compiling and Running Applications, Introduction to JVM
Datatypes and Variables
Primitive Datatypes, Declarations, Variable Names, Numeric Literals, Character Literals, Strings, StringBuffer, Arrays, Non-Primitive(reference) Datatypes
Operators and Expressions
Expressions, Assignment Operator, Arithmetic Operators, Relational Operators, Logical Operators, Increment and Decrement Operators, Shorthand Operators (+=, etc.), Conditional Operator, Operator Precedence, Implicit Type Conversions, The Cast Operator
Control Flow
Conditional Statements –if, switch, while and do-while Loops, for Loops, labels, The continue Statement, The break Statement
Object-Oriented Concepts
Introduction to Object-Oriented Programming, Classes and Objects, Fields and Methods, Encapsulation, Inheritance, Polymorphism, Access Modifiers
Inheritance in Java
Inheritance in Java, Constructors, Method Overriding, Super, this
Advanced Inheritance and Language Constructs
Enumerated Types, Abstract Classes, Interfaces, Generics
Inner Classes
Inner Classes, Member Classes, Local Classes, Anonymous Classes, Instance Initializers, Static Nested Classes
Exception Handling
Exceptions Overview, Catching Exceptions, The finally Block, Exception Methods, Declaring Exceptions, Defining and Throwing Exceptions, Errors and RuntimeExceptions, Assertions
Multi- Threading
Non-Threaded Applications, Threaded Applications, Creating Threads, Thread States, Runnable vs Thread, Synchronizing Threads
Input/Output Streams
Overview of Streams, Bytes vs. Characters, Converting Byte Streams to Character Streams, File Object, Binary Input and Output, PrintWriter Class, Reading and Writing Objects, Basic and Filtered Streams
Core Collection Classes
The Collections Framework, The Set Interface, Set Implementation Classes, The List Interface, List Implementation Classes, The Map Interface, Map Implementation Classes
Introduction to JDBC
The JDBC Connectivity Model, Creating a SQL Query, Getting the Results, Updating Database Data, Using a PreparedStatement, Parameterized Statements
Java Networking
Inet address, Socket, Server socket, URL, URL connection
Practical Sessions
Building and executing simple application using basic Java concepts – 1. Using multithreading in an application. 2. Using JDBC connectivity
JSP and Servlet Training
Introduction to Java Server Pages
What is JSP and why use it?, Embedding dynamic elements in HTML, Compilation, What you need to get started, Advantages
Servlet Basics
Request/Response model, Request parameters, Request methods, Servlet containers, Servlet Contexts and web applications
JSP Overview
Problem with Servlets, A Sample JSP Page in detail, JSP elements, JSP Application design with MVC architecture
Setting up the JSP Environment
Installing JSDK, Installing Tomcat Server (Web Server),Running sample example
Generation of Dynamic Content
Creating, installing and running a JSP page, Using JSP Directive elements, Using action elements, Using JSP standard tag library, Using Java Bean components in JSP pages - What is a bean?; Declaring bean in JSP; Retrieving bean properties in JSP page, Using Customer Tag Libraries - Overview of Custom tag library; Installing/declaring and using custom tags
Processing Input and Output
Reading request parameter values, Validating user input, Formatting the output
Error Handling and Debugging a JSP page
Handling Syntax errors, JSTL Expression Language errors, Debugging a JSP page, Handling runtime errors, Catching Exceptions
Sharing data between JSP, Requests and Users
Passing control and data between pages, Sharing session and application data, Accessing database, Memory usage considerations
Authentication and Personalization
Container – provided authentication, Application controlled authentication- Logging in; Authentication using database; Checking for a valid session; Updating user; Logging out; Security concerns
Internationalization
Locale Class, Formatting dates and numbers, Using localized text
Using Scripting Elements
Page Directive Scripting Attributes, Implicit scripting objects, Using scriptlets, expressions and declarations
Using JSP with an application model
J2EE Model – Brief overview, MVC Design Model, Using only JSP - JSP with Servlets; Servlets, JPS and EJB’s, Scalability – Distributed Development
Combining Servlets with JSP
Quick overview of Servlet lifecycle, Reading a request, Generating response, Using filters and listeners, Request processing using servlets, Using common JSP error page
Database Access Strategies
JDBC – Quick Overview, Using connection pools, Generic Database access bean
Practical Sessions:
Practicals will comprise of creating, installing, deploying and running a sample web application testing the usage of the following:
1.Developing Java Beans and its usage with JSP
2.Developing Custom Tag Libraries
3.Integrating Custom code with JSTL